Change Text Highlight Color with CSS
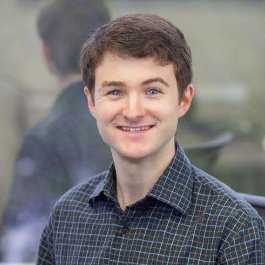
Braydon Coyer / October 20, 2020
2 minute read
•
1518 views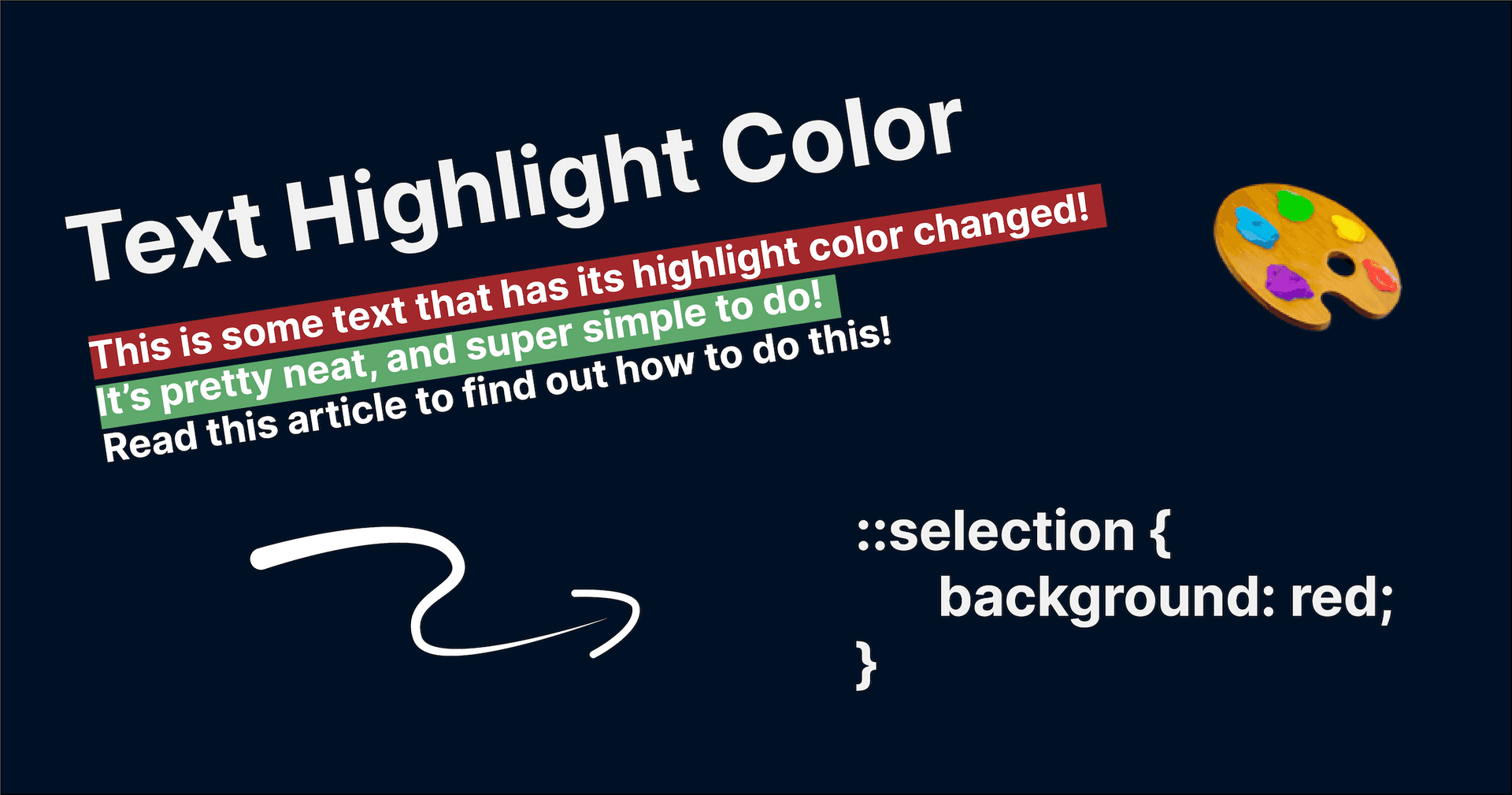
I’m pretty active on CodePen. A few months ago, I posted a pen that demonstrates how to change the highlight color of text on the page. I thought it may be neat to write up a short article that will walk you through the basics, and then go a bit deeper with CSS variables.
It’s pretty simple. To change the color of the highlighted-text, simply target the ::selection
selector and then define the color of the background
property.
Check out the snippet below.
1::selection {2 background: red;3}
Now that we know how easy it is to change the highlight color, let’s take it a step further and rotate colors by utilizing a bit of JavaScript and a single CSS variable.
First, in your JavaScript, define an array of strings - each item in the array is a hexadecimal color value.
Here’s my array:
1const colors = ["#c1b001", "#a8140e", "#4315aa", "#359d09", "#8f4762"];
We want to randomly assign a color from the array to our CSS variable when the user has their mouse button down to select some text.
In order to do this, we need to create a new event listener and listen for the mousedown
event.
Here’s my event listener:
1window.addEventListener("mousedown", () => {2 // code will go here3});
Now that we have this event set up, there’s three things we need to do:
- Grab and remove the first color from the array
- Set the CSS variable with the value we get back from the array
- Push the color variable from step 1 so it is now on the bottom of the array
This will gives us the rotating color functionality. Here’s my completed function:
1window.addEventListener("mousedown", (e) => {2 const color = colors.shift();3 document.documentElement.style.setProperty("--highlight-color", color);4 colors.push(color);5});
We’ve referenced a CSS variable named —highlight-color
but have not defined it yet.
In your CSS, define the variable and initialize it to null
.
1:root {2 --highlight-color: null;3}
Finally, target the ::selection
selector and set the background
property to the value in the CSS variable.
1::selection {2 background: var(--highlight-color);3}
And there we go! Here's the final pen - feel free to try it out!
Thanks for reading! If you liked this article and want more content like this, read some of my other articles and make sure to follow me on Twitter!
Articles delivered to your inbox!
A periodic update about my life, recent blog posts, how-tos, and discoveries.
As a thank you, I'll also send you a FREE CSS tutorial!
No spam - unsubscribe at any time!